Python
- Home
- »
- Python
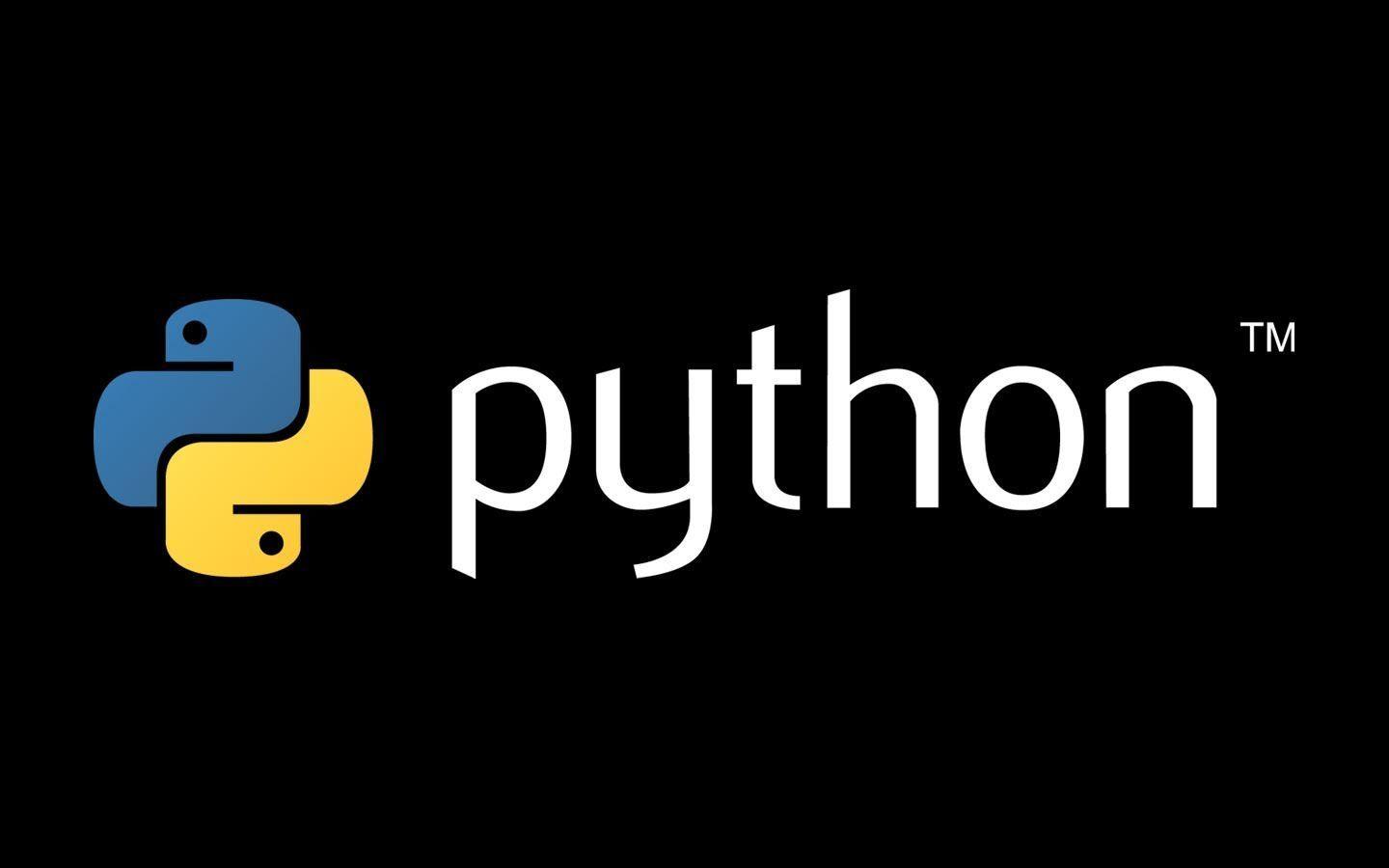
Python
- Whetting Your Appetite
- Using the Python Interpreter
- An Informal Introduction to Python
- More Control Flow Tools
- Data Structures
- Modules
- Input and Output
- Errors and Exceptions
- Classes
- Brief Tour of the Standard Library
- Brief Tour of the Standard Library — Part II
- Virtual Environments and Package
Topics Covered
Python
Data Structures
- More on Lists
- The del statement
- Tuples and Sequences
- Sets
- Dictionaries
- Looping Techniques
- More on Conditions
- Comparing Sequences and Other Types
Modules
- More on Modules
- Standard Modules
- The dir() Function
- Packages
Brief Tour of the Standard Library
- Operating System Interface
- File Wildcards
- Command Line Arguments
- Error Output Redirection and Program Termination
- String Pattern Matching
- Mathematics
- Internet Access
- Dates and Times
- Data Compression
- Performance Measurement
- Quality Control
- Batteries Included
Errors and Exceptions
- Syntax Errors
- Exceptions
- Handling Exceptions
- Raising Exceptions
- User-defined Exceptions
- Defining Clean-up Actions
- Predefined Clean-up Actions
Classes
- A Word About Names and Objects
- Python Scopes and Namespaces
- A First Look at Classes
- Random Remarks
- Inheritance
- Private Variables
- Odds and Ends
- Iterators
- Generators
- Generator Expressions
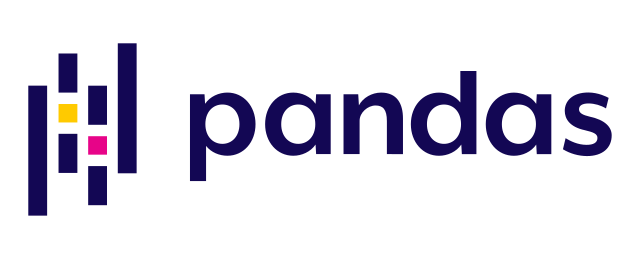

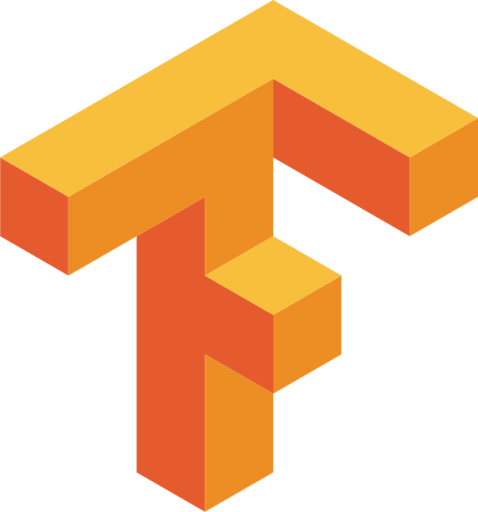


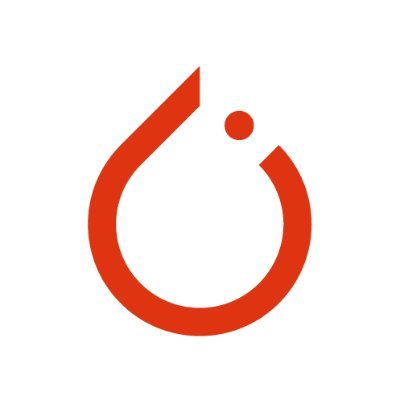
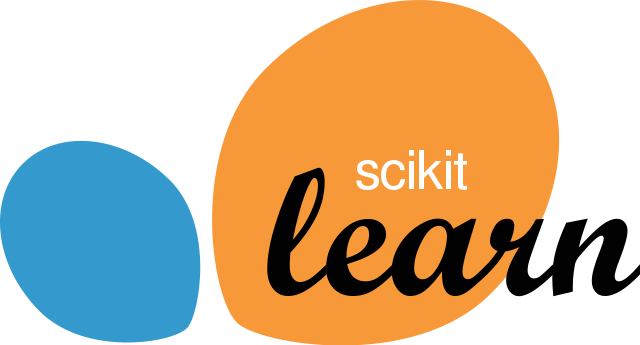
Brief Tour of the Standard Library — Part II
- Output Formatting
- Templating
- Working with Binary Data Record Layouts
- Multi-threading
- Logging
- Weak References
- Tools for Working with Lists
- Decimal Floating Point Arithmetic
Virtual Environments and Packages
- Introduction
- Creating Virtual Environments
- Managing Packages with pip
Copyright © 2024 IngeniousFusionTek | All Rights Reserved